JCheckBox is a Swing component that represents an item which shows a state of selected or unselected. User can change this state by clicking on the check box of the component. Here is a typical JCheckBox component in default Java look and feel:

A standard JCheckBox component contains a check box and a label that describes purpose of the check box. An icon and mnemonic key also can be set for this component.
Class Declaration
Following is the declaration for javax.swing.JCheckBox class −
public class JCheckBox
extends JToggleButton
implements Accessible
Field
Following are the fields for javax.swing.JCheckBox class −
static String BORDER_PAINTED_FLAT_CHANGED_PROPERTY − Identifies a change to the flat property.
Class Constructors
Sr.No. | Constructor & Description |
---|---|
1 |
JCheckBox()
Creates an initially unselected check box button with no text and no icon.
|
2 |
JCheckBox(Action a)
Creates a checkbox where the properties are taken from the Action supplied.
|
3 |
JCheckBox(Icon icon)
Creates an initially unselected checkbox with an icon.
|
4 |
JCheckBox(Icon icon, boolean selected)
Creates a checkbox with an icon and specifies whether or not it is initially selected.
|
5 |
JCheckBox(String text)
Creates an initially unselected checkbox with text.
|
6 |
JCheckBox(String text, boolean selected)
Creates a checkbox with the text and specifies whether or not it is initially selected.
|
7 |
JCheckBox(String text, Icon icon)
Creates an initially unselected checkbox with the specified text and icon.
|
8 |
JCheckBox(String text, Icon icon, boolean selected)
Creates a checkbox with text and icon, and specifies whether or not it is initially selected.
|
Class Methods
Sr.No. | Method & Description |
---|---|
1 |
AccessibleContext getAccessibleContext()
Gets the AccessibleContext associated with this JCheckBox.
|
2 |
String getUIClassID()
Returns a string that specifies the name of the L&F class which renders this component.
|
3 |
boolean isBorderPaintedFlat()
Gets the value of the borderPaintedFlat property.
|
4 |
protected String paramString()
Returns a string representation of this JCheckBox.
|
5 |
void setBorderPaintedFlat(boolean b)
Sets the borderPaintedFlat property, which gives a hint to the look and feel as to the appearance of the checkbox border.
|
6 |
void updateUI()
Resets the UI property to a value from the current look and feel.
|
Methods Inherited
This class inherits methods from the following classes −
- javax.swing.AbstractButton
- javax.swing.JToggleButton
- javax.swing.JComponent
- java.awt.Container
- java.awt.Component
- java.lang.Object
JCheckBox Example
Create the following Java program using any editor of your choice in say D:/ > SWING > com > sjavaspot > gui >
JCheckboxDemo.java
package com.sjavaspot.gui; import java.awt.*; import java.awt.event.*; import javax.swing.*; public class JCheckBoxDemo { private JFrame mainFrame; private JLabel headerLabel; private JLabel statusLabel; private JPanel controlPanel; public JCheckBoxDemo(){ prepareGUI(); } public static void main(String[] args){ JCheckBoxDemo jcheckBoxDemo = new JCheckBoxDemo();
jcheckBoxDemo.showCheckBoxDemo(); } private void prepareGUI(){ mainFrame = new JFrame("Java Swing Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); headerLabel = new JLabel("", JLabel.CENTER); statusLabel = new JLabel("",JLabel.CENTER); statusLabel.setSize(350,100); controlPanel = new JPanel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showCheckBoxDemo(){ headerLabel.setText("Control in action: CheckBox"); final JCheckBox chkApple = new JCheckBox("Apple"); final JCheckBox chkMango = new JCheckBox("Mango"); final JCheckBox chkPeer = new JCheckBox("Peer"); chkApple.setMnemonic(KeyEvent.VK_C); chkMango.setMnemonic(KeyEvent.VK_M); chkPeer.setMnemonic(KeyEvent.VK_P); chkApple.addItemListener(new ItemListener() { public void itemStateChanged(ItemEvent e) { statusLabel.setText("Apple Checkbox: " + (e.getStateChange()==1?"checked":"unchecked")); } }); chkMango.addItemListener(new ItemListener() { public void itemStateChanged(ItemEvent e) { statusLabel.setText("Mango Checkbox: " + (e.getStateChange()==1?"checked":"unchecked")); } }); chkPeer.addItemListener(new ItemListener() { public void itemStateChanged(ItemEvent e) { statusLabel.setText("Peer Checkbox: " + (e.getStateChange()==1?"checked":"unchecked")); } }); controlPanel.add(chkApple); controlPanel.add(chkMango); controlPanel.add(chkPeer); mainFrame.setVisible(true); } }
Compile the program using the command prompt. Go to D:/ > SWING and type the following command.
D:\SWING>javac com\sjavaspot\gui\JCheckboxDemo.java
If no error occurs, it means the compilation is successful. Run the program using the following command.
D:\SWING>java com.sjavaspot.gui.JCheckboxDemo
Verify the following output.
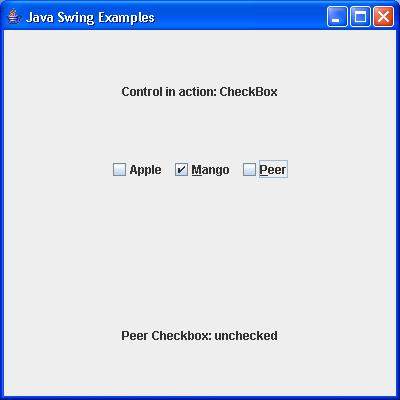
No comments:
Post a Comment